Bitsy Variables: A Tutorial
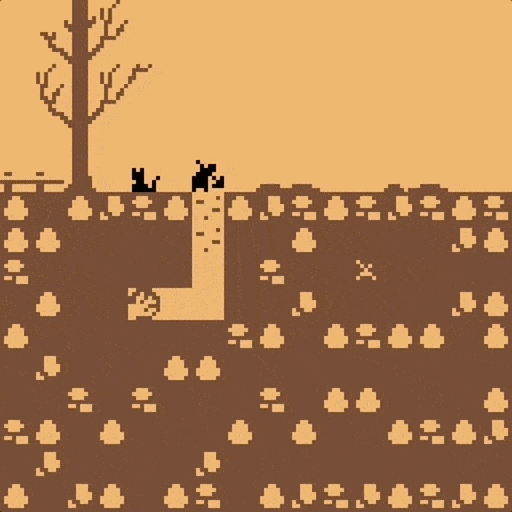
This is less a normal devlog and more of a tutorial on how to use variables in Bitsy. I’ve seen quite a bit of confusion about variables from folks trying to learn Bitsy. Right now there’s no real Bitsy documentation, so I figured I could share what I’ve been able to figure by myself about them. I’ll use parts of this game as examples, however, so ! ! SPOILER ALERT ! ! if you’re worried about spoilers for a 20min Bitsy game.
NOTE: This tutorial is written for Bitsy 4.6. It is more than likely that some of this tutorial may change or become obsolete in the future. I will try to update it as Bitsy does.
I’m going to be writing this tutorial assuming my audience has little to no other programming experience. There’s a TL;DR at the bottom if you don’t need any introduction to programming concepts and just want a cliff-notes version.
O K 👏L E T S 👏 L E A R N 👏B I T S Y 👏V A R I A B L E S
The Basics:
If you don’t know what a variable is at all, it’s a piece of data your game can store and use to perform logical operations. Basically if you want your Bitsy project to be less story (a linear narrative all players will encounter in the same way) and more game (a scenario where player choices affect what occurs), you’re going to need to use variables. This tutorial is less about why/when you should use variables, and more about the literal how/what.
Here’s the first, most basic things you need to know about variables in Bitsy:
- You can define a variable from the ‘inventory’ window of the Bitsy editor.
- Variables can be used in conditionals from the dialog window of the Bitsy editor.
- Variables can be set from inside dialog using curly braces and the equals sign:
{myVariable = 1}
{myVariable = myVariable - 6}
myVariable is equal to {say myVariable}
This is probably a good time to mention curly braces. In case you don’t know, curly braces ‘{}’ in Bitsy dialog represent a ‘code block.’ Meaning that Bitsy knows that when it encounters an open curly brace, that means that everything between that and the corresponding close curly brace are code it needs to process, rather than simply text it needs to display.
Pro-tip: Get comfortable with editing dialog using the ‘show code’ option. There’s some things the code can do that you just can’t otherwise. Also, as your dialog logic gets more complicated, the code view will be much faster to read.
Real World Example:
This little snippet of scripting contains just about everything I know about variables in Bitsy. This is (a simplified version of) the dialog for the cat Bernice in OTTFHS.
{ - metBernice >= 1 ? - else ? {bernices = bernices + 1} {metBernice = 1} }{ - bernices == 1 ? {tempString = ":"} - else ? {tempString = " " + bernices + ":"} }Bernice{say tempString} {wvy}mrowwwr.{wvy}
In plain english, here’s what it does: It checks to see if this is the first time you’ve met Bernice, and if it IS, it adds one to the number of Bernices you’ve found. Then, if you’ve found more than one Bernice, it puts a space and a number after her name in the dialog. Then she meows at you.
In case it helps, that code looks like this in the editor GUI:
And then here’s what that looks like in-game:
Looking back at the code for that dialog, you might see where I set a variable’s value in that code snippet:
{metBernice = 1}
You can also see where I had a variable display in the dialog:
{say tempString}
I also added to a variable's existing value:
{bernices = bernices + 1}
PRO-TIP: Although each item in the game has an associated value that tracks how many of them you have picked up, you will rarely be able to do everything you’d like to with that value. It’s much wiser to instead define a variable that is associated with that item and is added to every time you pick one of those items up.
Slightly More Advanced Variables:
{tempString = ":"}
So, this block in that snippet is important because I’m doing two things with variables we haven’t discussed yet. I’m defining a variable from dialog, and I’m storing a string as a variable. Let’s tackle those one at a time.
tempString is not a variable I had defined previous to that code block. It works exactly like any other variable in Bitsy. You can even see it in the variables window of your editor after it’s been defined if you are previewing your game from the editor. Why would I do this? Well, I needed a quick variable just for this dialog snippet and not anywhere else. I COULD define that variable in the editor, but that would just clutter things up. I have no plan to use it again, so I just define it on the fly. Even though these variables are identical to all other variables in Bitsy, I would highly recommend for your own sanity, you ONLY use this to define temporary variables.
Pro-tip: It is a very good practice to name your variables things that tell you what they do. A variable named ‘g’ works just as well as one named ‘playerGold,’ but one of them will make debugging problems later MUCH easier.
Variables in Bitsy can be either numbers or strings. I imagine you know what a number is, but in case you’re wondering what a string is, it’s a string of text characters. Think a word or a sentence. This can be used in a lot of handy ways. In the case above, tempString is storing a single colon character. I’m using it to format Bernice’s name and number correctly. In order to define a variable as a string, set it equal to whatever characters you want, contained within quotation marks.
{stringVar = "foo bar!!"}
Bitsy can perform one (and only one) operation on strings. You can ‘add’ or concatenate strings together using the plus symbol.
{stringVar = "foo" + "bar"}
Just to give you a better idea of how to use strings, and why they’re important, here's another example. In OTTFHS, after you find the Truck, you learn Trevor’s Grandpa’s name is Steve. I wanted that to be reflected in dialog, but I didn’t want to restrict the player to finding items in a certain order. So, I defined a variable ‘grandpaName’ , set its initial value to “Gramps” , and then when the player found the Truck item, I set it to ‘Granda Steve’. Whenever talking to Grandpa, I start his dialog by outputting that variable using ‘{say grandpaName}’
Before we find the truck:
And afterwards:
Final Thought: Store Endings as Strings
Currently in Bitsy, ‘say’ is the only scripting that is valid in title and ending text. This can make creating branching endings a little hard to create, since each possible game ending needs to be placed on a different tile. You can’t just have one ending and display different text based on a conditional. The work-around for this currently is to store your ending text as a variable. You can change the ending text via dialog whenever you need to, and then simply have your ending display whatever string you have stored in your variable.
Alright! That's pretty much all all I know about variables in Bitsy currently. 😉Hopefully this helps folks make awesome Bitsy projects!
TL;DR
Bitsy variable quick-reference:
- ‘say’ prints a variable in dialog.
- It is best practice to favor using variables over relying on item counts.
- You can declare new variables on the fly, and all variables in Bitsy are global
- Variables in Bitsy are either Numbers or Strings.
- Define strings with double quotes only. No single quotes!
- Available operators are = + - / * (Only = and + are meaningful for strings)
- Math operators cannot be used in a conditional. Each conditional can only evaluate one variable.
- ‘say’ is the only scripting allowed in titles/endings: consider storing/editing ending text as a string
Files
On Tuesday, Trevor Found His Shovel
A Bitsy game about digging up the past. Made for the Archaelogy bitsyjam.
Status | Released |
Author | AYolland |
Genre | Interactive Fiction |
Tags | archaeology, Bitsy, bitsyjam |
Comments
Log in with itch.io to leave a comment.
Is it possible to edit the text with tags such as {wvy} in a string's content?
hey! I know this is 2 years old, BUT it interested me enough to go ask Bitsy's creator! here's the solution he proposed:
Thank you for this! Was confused when I saw variables in the editor and nothing explaining how to use them.
Amazing! Thank you so much! Is there any place where a random number could be created and used as a variable?
(oh hey, love your art!)
Without hacks, you’ll need to use the ‘shuffle’ list function. Not elegant, but it works. You’ll have to write a `{variable = X}` for each possible value, and repeat that whole list wherever you you need another similar random number. If that gets unwieldy, you’re probably better off using the ‘arbitrary JavaScript’ hack and writing your own RNG function. Definitely an easy script to find/write if you go that route.
thanks for the kind words! I really, really loved OTTFHS!! So so pretty and mysterious.
Hm I suspected that would be the case! Sounds easy enough! I need to make a throwaway sprite and give it a list of variables, and touch it with my avatar whenever I want a random number, correct?
The only other thing I would like in bitsy is the ability to have a larger avatar. Even 16x16 would be an improvement. Have you, without hacks, found a way to make it true? Like, sprites following the avatar or something.
Thank you for the explanation <3
You could make a sprite that saves a random number to a variable. Or more likely, you could include that same dialog that generates the number in the middle of whatever dialog where it's required.
As far as 16x16 sprites would go, it's not possible for the player avatar to be more than a single 8x8 sprite without hacks. (There is a hack for that.) Some people have created vanilla Bitsy games that create an illusion of a larger avatar using exits, but that has limits. You can of course have a game with an 8x8 avatar but with larger, multisprite characters that they interact with.
you're amazing, thank you!!!! If you ever want a collab, I'm kinda good with 1-bit graphics. So long as they're larger than 8x8 ^-^'
Awesome! I had a basic understanding of Bitsy variables but did realise just what could be done with em! Thanks for the tutorial.
Great tutorial - I thought I knew Bitsy variables reasonably well, but I still learned a lot.
whoa this is really cool! i hadnt ever thought you could use variables like this.. guess i might attempt to try soon :D